A Simple Guide to APIs (Duck Puns included)
Hello, fellow tech enthusiasts and duck aficionados! Today, we’re going to waddle into the wonderful world of APIs. Now, before you start flapping your wings in confusion, let me assure you: this guide will be as simple as a duck’s life on a sunny pond and, hopefully, twice as fun. So, let’s dive in, beak first, into understanding API’s without ruffling too many feathers!
What Exactly is an API?
API stands for Application Programming Interface.
Imagine an API as a friendly duck waiter at your favorite pond-side café. You’re sitting at your table (your application), and you want some delicious data (like a crispy bread crumb or a piece of cake). The waiter (the API) takes your order to the kitchen (the server), fetches what you need, and brings it back to you.
Why Do We Need APIs?
Just like ducks need water, applications need APIs to interact with each other. APIs allow different software applications to communicate, share data, and work together without needing to know each other’s secret recipes. Without APIs, every time you wanted to do something like check the weather, send a tweet, or stream a video, you’d have to reinvent the wheel.
Types of APIs: A Duck’s Guide
APIs come in all shapes and sizes, much like ducks! Let’s break down the main types:
- Web APIs: These are the most common and are used to interact with web-based services. Think of them as the mallards of the API world. They’re everywhere!
- Library APIs: These APIs allow different pieces of software to talk to each other within the same environment. They’re like a family of ducks quacking together in the same pond.
- Operating System APIs: These help software communicate with the operating system. Imagine these as ducks that help your computer’s brain tell its body how to waddle.
- Database APIs: These let applications interact with databases, fetching and storing data like a duck foraging for tasty bugs.
How Do APIs Work?
To understand how APIs work, let’s follow the journey of a simple API request:
- Request: Your application sends a request to an API. It’s like sending a little duckling off to fetch something for you.
- Endpoint: This request is sent to an API endpoint, a specific address where the API waits for requests. Think of it as a duck’s nest, cozy and ready to receive.
- Method: Your request includes a method, such as GET (fetch data) or POST (send data). It’s like telling your duckling what exactly to bring back or deliver.
- Response: The API processes your request and sends back a response, much like our duckling returning with a beakful of goodies.
- Data: The data is then delivered to your application, ready to be used or displayed. It’s like getting that tasty breadcrumb you were waiting for!
Real-Life Duck (API) Tales
Let’s dip our toes into some real-life examples of APIs making waves in the tech pond.
Weather Forecasting
Ever wondered how weather apps dish out those accurate forecasts? They use APIs! When you open your weather app and check if it’s going to rain, the app sends a request to a weather API. The API responds with the latest weather data, and voilà, you know whether to bring an umbrella or not.
Social Media Integration
Quacking about your latest adventure on social media? APIs are the ones making it happen! When you post a picture on Instagram and share it on Twitter simultaneously, APIs are busy working behind the scenes, ensuring everything syncs up smoothly.
Payment Gateways
Ever bought something online? You have APIs to thank for that seamless transaction. Payment gateways use APIs to process your payment securely, communicating with your bank and the merchant without you having to worry about a thing.
The Anatomy of an API Call: Step by Step
Let’s dissect an API call to understand its anatomy better. We’ll use the example of a web API that fetches information about different types of ducks (because why not?).
Step 1: The Request
Your application sends an HTTP request to the API. Here’s what a typical request might look like:
http
GET /api/ducks HTTP/1.1
Host: duckapi.com
Authorization: Bearer YOUR_ACCESS_TOKEN
- GET: This is the HTTP method, telling the API we want to retrieve data.
- /api/ducks: This is the endpoint, specifying that we want information about ducks.
- Authorization: This is where you include any necessary access tokens to authenticate your request.
Step 2: The Response
The API processes your request and responds with data, usually in JSON format:
json
{
"ducks": [
{
"name": "Mallard",
"color": "Green",
"habitat": "Freshwater ponds"
},
{
"name": "Pekin",
"color": "White",
"habitat": "Domestic"
}
]
}
- “ducks”: This is a collection of duck data.
- Each duck object contains information such as name, color, and habitat.
Authentication and Security: Keeping Your Pond Safe
Just like a duckling needs protection from predators, your API needs protection from unauthorized access. Here are some common methods used to secure APIs:
API Keys
An API key is a unique identifier that you include in your request to authenticate yourself. Think of it as a special quack that only you know.
OAuth
OAuth is a more advanced authentication method that allows third-party applications to access your data without exposing your credentials. It’s like having a trusted duck friend who can vouch for you.
Rate Limiting
To prevent abuse, APIs often enforce rate limits, restricting the number of requests you can make in a given time period. It’s like making sure no one duck hogs all the bread crumbs.
Building Your Own API: A Duckling’s First Swim
So, you want to build your own API? That’s fantastic! Here’s a high-level overview to get you started on your first swim:
Step 1: Define Your Purpose
What will your API do? Will it fetch data about different breeds of ducks, or perhaps manage a collection of duck photos? Define the scope and functionality of your API.
Step 2: Choose Your Tools
Select the technologies you’ll use. Common choices include:
- Server: Node.js, Python (Flask/Django), Ruby on Rails.
- Database: MySQL, PostgreSQL, MongoDB.
- Framework: Express (for Node.js), Flask/Django (for Python).
Step 3: Design Your Endpoints
Plan your API endpoints. For instance, if you’re building a duck information API, you might have endpoints like:
- GET /ducks: Retrieve a list of all ducks.
- POST /ducks: Add a new duck.
- GET /ducks/: Retrieve information about a specific duck.
Step 4: Implement Authentication
Decide how you’ll secure your API. Will you use API keys, OAuth, or another method? Implement the necessary authentication mechanisms.
Step 5: Write Your Code
Start coding your API, defining the endpoints and their functionalities. Ensure you handle errors gracefully and provide meaningful responses.
Step 6: Test, Test, Test
Thoroughly test your API to make sure it works as expected. Use tools like Postman or curl to send requests and verify the responses.
Step 7: Documentation
Write clear documentation for your API so that other developers know how to use it. Include examples, endpoint descriptions, and authentication details.
Common API Protocols: Ducks of a Different Feather
Different APIs use different protocols to communicate. Here are the most common ones:
REST (Representational State Transfer)
REST is the mallard of API protocols – ubiquitous and reliable. It uses standard HTTP methods and is designed around resources, such as ducks, identified by URLs.
SOAP (Simple Object Access Protocol)
SOAP is a bit more complex, like a rare duck species. It uses XML for messaging and is known for its robustness and security features.
GraphQL
GraphQL is a relatively new duck on the pond. It allows clients to request exactly the data they need, no more, no less, which can make it more efficient than REST in some cases.
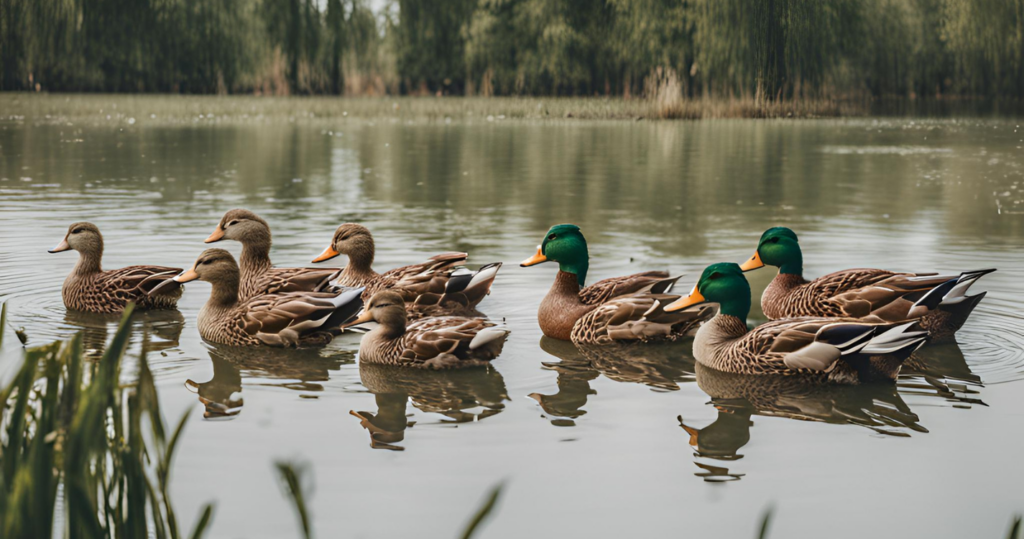
API Best Practices: Swimming Smoothly
To ensure your API swims smoothly and keeps developers happy, follow these best practices:
Keep It Simple
Simplicity is key. Make sure your API is easy to understand and use. Avoid overcomplicating things – remember, even a duckling should be able to use it!
Provide Clear Documentation
Good documentation is like a map for other developers. Include clear examples, endpoint descriptions, and any necessary authentication details.
Version Your API
APIs evolve over time. Use versioning to manage changes without breaking existing applications. For example, use URLs like /api/v1/ducks and /api/v2/ducks.
Handle Errors Gracefully
Provide meaningful error messages and status codes. If something goes wrong, developers should know what happened and how to fix it.
Ensure Security
Protect your API with proper authentication and encryption. Nobody likes unauthorized ducks in their pond!
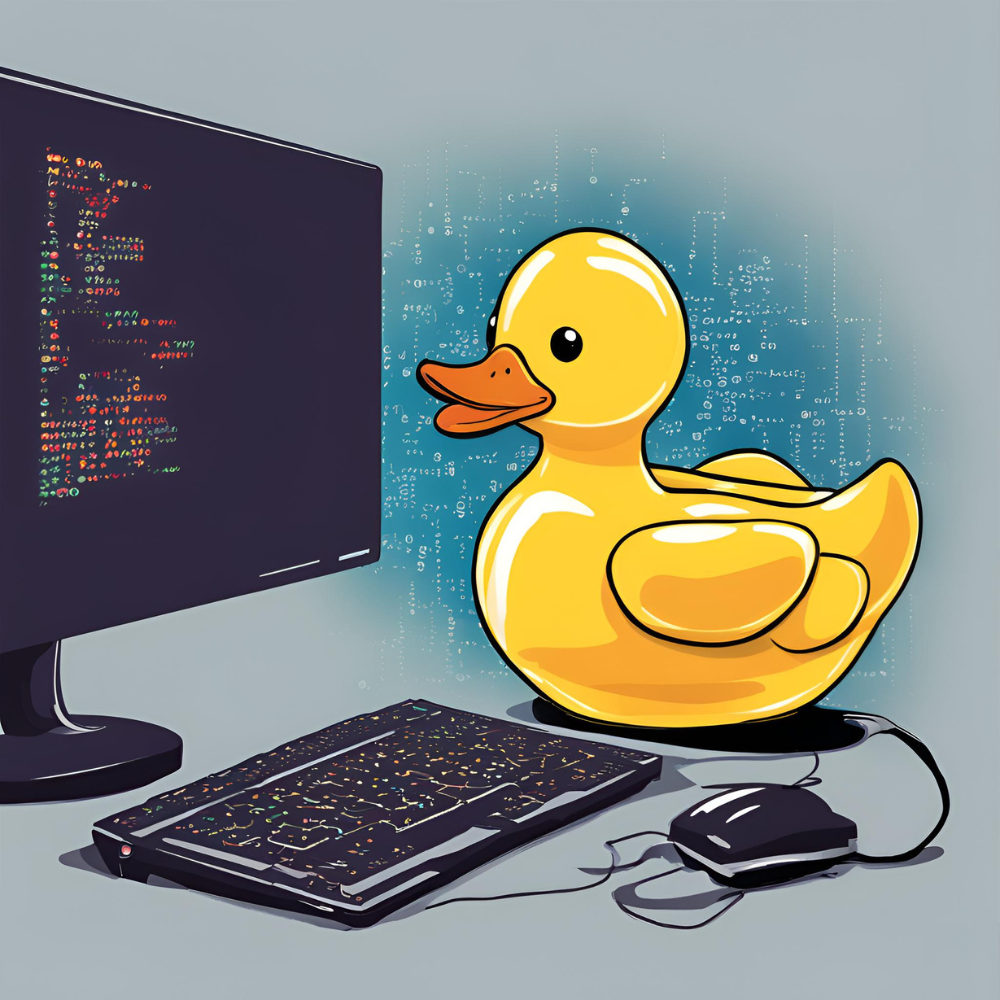
Create your own API with our free-to-use tool! We simplified the whole process of building an API from scratch. All you have to do is fill out a form and the tool will create a fully functional API for you.